Operators in Python
What is Operator ?
Operator in Python is a symbol which is used to perform operations. For example: +, -, *, / etc.

Here + is Operator and 10,20 are the operands and 10+20 is an Expression.
What is Operand in Python?
The value given to the operator for operation is known as Operand in Python.
What is Expression in Python?
The combination of Operator and Operands is Known as Expression in Python.
Types of Operators in Python.
1. Binary Operators. 2. Assignment Operators. 3. Membership Operators.
Binary Operators:
The operator which works on two operands.
Assignment Operator :
The operator which assigns a value from Right to Left.
Membership Operators:
The operators which are used to test the membership of a value in Strings etc.
Binary Operators:

Arithmetic Operators
Arithmetic Operators are the operators which perform arithmetic calculations.
In the following table, the value of 'a' is 8 whereas that of 'b' is 4.

Addition(+): This operator is used to get the sum of 2 values.
Example: x=2,y=5
x+y
Output
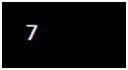
Subtraction(-): This operator is used to get the difference of 2 values.
Example: x=8,y=5
x-y
Output
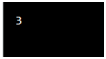
Multiplication(*): This operator is used to get the multiplication of 2values.
Example: x=2,y=5
x*y
Output
Modulus(%): This operator is used to get the remainder of 2 values.
Example: x=3,y=2
x%y
Output
Exponentiation(**): This operator is used to get the exponent power of a variable.
Example: x=2,y=5
x**y is same as x^y
Output
Division(/): This operator is used to get the rounded whole number from division of 2 values.
Example: x=8, y=5
x/y
Output
Floor division(//): This operator is used to get the rounded whole number from division of 2 values.
Example: x=8, y=5
x//y
Output
Relational Operators
Relational operators in Python return True if the relationship is True and False if the relationship is False.
In the following table, assume the value of 'a' to be 8 and that of 'b' to be 4
Double Equal to(==): This operator is used to compare the values of 2 variables and return True if both values are equal, else return false.
Example: x=3,y=3
x==y
Output
Not Equal to(!=): This operator is used to compare the values of 2 variables and return True if both values are not equal, else return false.
Example: x=3,y=4
x!=y
Output
Greater than(>): This operator is used to compare the values of 2 variables and return True if the first value is greater than the second, else return false.
Example: x=4,y=2
x>y
Output
Less than(<): This operator is used to compare the values of 2 variables and return True if the first value is smaller than the second, else return false.
Example: x=4,y=2
x<y
Output
Greater than or Equal to(>=): This operator is used to compare the values of 2 variables and return True if the first value is greater than or equal to the second, else return false.
Example: x=4,y=4
x>=y
Output
Less than or Equal to(<=): This operator is used to compare the values of 2 variables and return True if the first value is smaller than or equal to the second, else return false.
Example: x=3,y=2
x<=y
Output
Logical Operators.
A logical operator in Python is an operator that returns a Boolean result. (true or false)
and operator: This operator returns True if both the conditions are true.
Example: x=4,y=3
x>y and y==3
Output
or operator: This operator returns True even if one of the conditions are true.
Example: x=2,y=3
x>y or y==3
Output
not operator: This operator reverses the result.
Example: x=4,y=3
not(x>y and y==3)
Output
Assignment Operator
Assignment operator assign values from its right side to its left side.For example, a = 5 assigns a value of 5 to the variable 'a'.
Single Equal to(=): This operator is used to assign the value to a variable.
Example: x=5
Plus Equal to(+=): This operator is used to add the new value to the previous value of a variable and assign the updated value.
Example: x=5
x+=3
Or
x=x+3
Output
Minus Equal to(-=): This operator is used to subtract the new value from the previous value of the variable and assign the updated value.
Example: x=7
x-=6
Or
x=x-6
Output
Multiplication Equal to(*=): This operator is used to multiply the new value from the previous value of the variable and assign the updated value.
Example: x=2
x*=2
Or
x=x*2
Output
Divide Equal to(/=):This operator is used to divide the new value from the previous value of the variable and assign the updated value.
Example: x=8
x/=4
Or
x=x/4
Output
Modulus Equal to(%=):This operator is used to divide the new value from the previous value of the variable and assign the remainder.
Example: x=5
x%=3
or
x=x%3
Output
Floor Division Equal to(//=):This operator is used to divide the new value from the previous value of the variable and assign the whole number of the division.
Example: x=17
x//=2
Or
x= x//2
Output
Exponentiation Equal to(**=): This operator is used to calculate the power and assign the updated value.
Example: x=5
x**=2
Or
x=x**2
Output
Membership Operators:
These are used to test if a sequence is present in an object.
in: This operator returns True if a sequence is present in the object.
Example: x=[2,3,4]
print(2 in x)
Output
not in: This operator returns True if a sequence is not present in the object.
Example: x=[2,3,4]
print(8 not in x)
Output
Identity Operators:
These are used to compare 2 variables i.e if they are same object with same memory location or not.
is: This operator returns True if both the variables are same object.
Example: x=3
y=x
x is y
Output
is not: This operator returns True if both the variables are not the same object.
Example: x=3
y=x
x is not y
Output