Java Swing JCheckBox
Java Swing JCheckBox
The JCheckBox class is the implementation of check boxes in swings. A checkbox allows the user to turn something on and off.CheckBoxes are used to provide multiple options to the user,from which user can select more than one opetions.It inherits JToggleButton class.
Constructors used with JCheckBox
Type of Constructor | Constructor Description |
---|---|
public JCheckBox( ) | Creates a checkbox without text and icon. The state of the checkbox is not selected. |
public JCheckBox(Icon icon) | Creates a checkbox with icon |
public JCheckBox(Icon icon, boolean selected) | Creates a checkbox with icon and initialize the state of checkbox by the boolean parameter selected |
public JCheckBox(String text) | Creates a checkbox with text |
public JCheckBox(String text, boolean selected) | Creates a checkbox with text and initialize the state of the checkbox |
public JCheckBox(String text, Icon icon) | Creates a checkbox which displays both text and icon. |
public JCheckBox(String text, Icon icon, boolean selected) | Creates a checkbox which displays both text and icon. The state of the checkbox can be initialized. |
The following example create an object of class JCheckBox.
Example
Basic Methods of JCheckBox class :
Method Name | Description |
---|---|
String getLabel() void setLabel(String) | Sets or gets a label for a check box. |
boolean getState() void setState(boolean) | Sets or gets the status of the check box. |
Example of JCheckBox with JCheckBox() and JCheckBox(String, boolean)
import javax.swing.*;
class CheckBoxEx extends JFrame
{
JCheckBox chk1,chk2,chk3;
JLabel lbl;
public CheckBoxEx()
{
chk1=new JCheckBox();
chk1.setText("C++");
chk2=new JCheckBox("Java ",true);
chk3=new JCheckBox("Python");
lbl=new JLabel("Select any course :");
lbl.setBounds(50, 30, 100, 30);
chk1.setBounds(30, 70, 50, 30);
chk2.setBounds(90, 70, 70, 30);
chk3.setBounds(170, 70, 80, 30);
add(lbl);
add(chk1);
add(chk2);
add(chk3);
add(chk1); add(chk2); add(chk3);
setLayout(null);
setSize(350,300);
setTitle("Check Boxes Example");
setVisible(true);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
}
}
public class CheckBoxExample
{
public static void main(String[] args)
{
CheckBoxEx checkbox=new CheckBoxEx();
}
}
The code above will display :
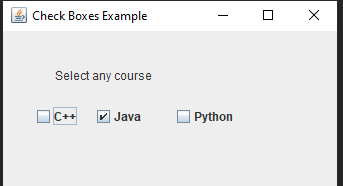
Example of Java Swing JCheckBox with ActionListener
import javax.swing.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
class CheckBoxEx extends JFrame implements ActionListener
{
JCheckBox chk1,chk2,chk3;
JLabel lbl;
JButton btn;
String msg="";
int fee=0;
public CheckBoxEx()
{ chk1=new JCheckBox();
chk1.setText("C++ @ 5000/-");
chk2=new JCheckBox("Java @ 6000/-");
chk3=new JCheckBox("Python @ 7000/-");
lbl=new JLabel("Select Course: ");
btn=new JButton("Click the Course");
lbl.setBounds(80, 50, 300, 30);
chk1.setBounds(80, 100, 150, 30);
chk2.setBounds(80, 130, 150, 30);
chk3.setBounds(80, 160, 150, 30);
btn.setBounds(80, 200, 150, 30);
add(lbl);
add(chk1);
add(chk2);
add(chk3);
add(chk1); add(chk2); add(chk3);
add(btn);
btn.addActionListener(this);
setLayout(null);
setSize(350,300);
setTitle("Check Boxes Example");
setVisible(true);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
}
public void actionPerformed(ActionEvent ae)
{
if(chk1.isSelected())
{
msg="C++: 5000\n";
fee+=5000;
}
if(chk2.isSelected())
{
msg+="Java: 6000\n";
fee+=6000;
}
if(chk3.isSelected())
{
msg+="Python: 7000\n";
fee+=7000;
}
msg+="-----------------\n";
JOptionPane.showMessageDialog(this,msg+"Total: "+fee);
}
}
public class CheckBoxExample
{
public static void main(String[] args)
{
CheckBoxEx checkbox=new CheckBoxEx();
}
}
The code above will display :
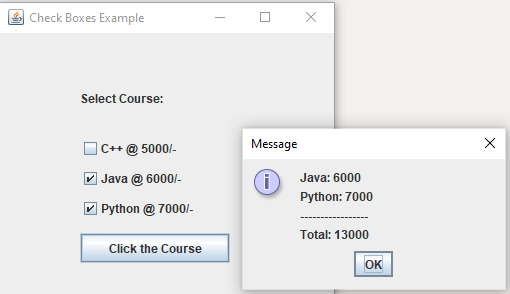
Example of Java Swing JCheckBox with ItemListener
import javax.swing.*;
import java.awt.event.ItemEvent;
import java.awt.event.ItemListener;
class CheckBoxEx extends JFrame implements ItemListener
{
JCheckBox chk1,chk2,chk3;
JLabel lbl;
String msg="";
public CheckBoxEx()
{
chk1=new JCheckBox();
chk1.setText("C++");
chk2=new JCheckBox("Java");
chk3=new JCheckBox("Python");
lbl=new JLabel();
lbl.setBounds(50, 30, 300, 30);
chk1.setBounds(30, 70, 50, 30);
chk2.setBounds(90, 70, 70, 30);
chk3.setBounds(170, 70, 80, 30);
add(lbl);
add(chk1);
add(chk2);
add(chk3);
add(chk1); add(chk2); add(chk3);
chk1.addItemListener(this);
chk2.addItemListener(this);
chk3.addItemListener(this);
setLayout(null);
setSize(350,300);
setTitle("Check Boxes Example");
setVisible(true);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
}
public void itemStateChanged(ItemEvent ie)
{
if(ie.getSource()==chk1)
{
msg += chk1.getText()+" ";
}
if(ie.getSource()==chk2)
{
msg += chk2.getText()+" ";
}
if(ie.getSource()==chk3)
{
msg += chk3.getText()+" ";
}
lbl.setText("You have selected :"+msg);
}
}
public class CheckBoxExample
{
public static void main(String[] args)
{
CheckBoxEx checkbox=new CheckBoxEx();
}
}
The code above will display :
