Java Swing JList
Java Swing JList
To create list we use JList class.JList class object shows the list of items.A list allows user to choose either a single selection or multiple selections.
The object of JList class represents a list of text items. The list of text items can be set up so that the user can choose either one item or multiple items.A listModel which is an interface is used for maintaining the contents
of the list. A class DefaultListModel is used for maintaining a contents list.The class DefaultListModel inherits ListModel.
Constructors used with JList
Type of Constructor | Constructor Description |
---|---|
public JList( ) | It creates an empty list. |
public JList(Object[] arr) | Creates a JList that displays the elements in the specified array. |
public JList(ListModel listModel) | It creates a list from a given model. |
public JList(Vector<?> vector) | Creates a list that displays elements of a given vector. |
The following example create an object of class JList.
Example
Basic Methods of JList class :
Method Name | Description |
---|---|
getSelectedValue() | It returns the selected value of the element of the list. |
getSelectedIndex() | It returns the index of selected item of the list |
setSelectionBackground(Color c) | It sets the background Color of the list. |
setSelectionForeground(Color c) | It Changes the foreground color of the list |
setSelectedIndex(int x) | It sets the selected index of the list to x |
setVisibleRowCount(int c) | It changes the visibleRowCount property |
isSelectedIndex(int i) | It returns true if the specified index is selected, else false. |
setLayoutOrientation(int o) | It defines the orientation of the list |
setFixedCellHeight(int h) | It changes the cell height of the list to the value passed as parameter. |
setFixedCellWidth(int w) | It changes the cell width of list to the value passed as parameter. |
Example of JList with JList(Object[] arr)
import javax.swing.*;
import java.awt.*;
class ListEx extends JFrame
{
JLabel lbl;
JList li;
public ListEx()
{
String course[]={"C","C++","Java","Python","HTML","CSS","JavaScript","PHP"};
lbl=new JLabel("Select Course: ");
li=new JList(course);
li.setSelectedIndex(3);
lbl.setBounds(40, 40, 80, 40);
li.setBounds(40, 80, 80, 150);
add(lbl);
add(li);
setLayout(null);
setSize(300,300);
setTitle("Radio Button");
setVisible(true);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
}
}
public class ListExample
{
public static void main(String[] args)
{
ListEx list=new ListEx();
}
}
Output
The code above will display :
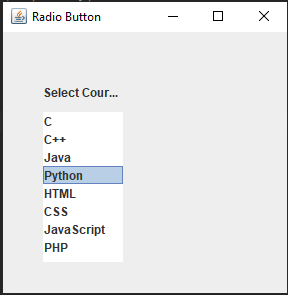
Example of Java Swing JList with DefaultListModel<String> and use of setSelectionInterval() method
import javax.swing.*;
import java.awt.*;
{
JLabel lbl;
JList li;
public ListEx()
{
DefaultListModel<String> sports = new DefaultListModel<>();
sports.addElement("Tennis");
sports.addElement("Archery");
sports.addElement("Cricket");
sports.addElement("Hockey");
sports.addElement("Rugby");
sports.addElement("Football");
int begn = 0;
int end = 3;
lbl=new JLabel("Select Course: ");
li=new JList(sports);
lbl.setBounds(40, 40, 100, 40);
li.setBounds(40, 80, 80, 120);
add(lbl);
add(li);
li.setSelectionInterval(begn, end);
setLayout(null);
setSize(300,300);
setTitle("Radio Button");
setVisible(true);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
}
}
public class ListExample
{
public static void main(String[] args)
{
ListEx list=new ListEx();
}
}
Output
The code above will display :
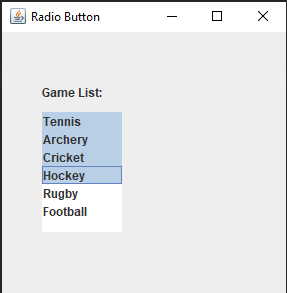
Example of Java Swing JList of adding items using Loop:
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
class ListEx extends JFrame
{
JLabel lbl;
JList li;
public ListEx()
{
final int MAX = 5;
String[] listElems = new String[MAX];
for (int i = 0; i < MAX; i++) {
listElems[i] = "element is : " + (i+1);
}
lbl=new JLabel("Add items Using Loop ");
li=new JList(listElems);
lbl.setBounds(40, 40, 100, 40);
li.setBounds(40, 80, 80, 120);
add(lbl);
add(li);
setLayout(null);
setSize(400,300);
setTitle("Radio Button");
setVisible(true);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
}
}
public class ListExample
{
public static void main(String[] args)
{
ListEx list=new ListEx();
}
}
Output
The code above will display :

Example of Java Swing JList with ActionListener
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
class ListEx extends JFrame implements ActionListener
{
JLabel lbl;
JList li;
JButton btn;
public ListEx()
{
DefaultListModel<String> course = new DefaultListModel<>();
course.addElement("C");
course.addElement("C++");
course.addElement("Java");
course.addElement("Python");
course.addElement("JavaScript");
course.addElement("PHP");
lbl=new JLabel("Select Course: ");
li=new JList(course);
btn=new JButton("Click");
lbl.setBounds(40, 40, 100, 40);
li.setBounds(40, 80, 80, 120);
btn.setBounds(150, 120, 100, 30);
add(lbl);
add(li);
add(btn);
btn.addActionListener(this);
setLayout(null);
setSize(400,300);
setTitle("Radio Button");
setVisible(true);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
}
public void actionPerformed(ActionEvent ae)
{
String msg = "";
if (li.getSelectedIndex() != -1) {
msg = "You have selected " + li.getSelectedValue()+" Programming language.";
}
JOptionPane.showMessageDialog(this,msg);
}
}
public class ListExample
{
public static void main(String[] args)
{
ListEx list=new ListEx();
}
}
Output
The code above will display :

Example of Java Swing JList with ActionListener using getSelectedIndices() and getModel() methods
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
class ListEx extends JFrame implements ActionListener
{
JLabel lbl;
JList li;
JButton btn;
public ListEx()
{
DefaultListModel<String> course = new DefaultListModel<>();
course.addElement("C");
course.addElement("C++");
course.addElement("Java");
course.addElement("Python");
course.addElement("JavaScript");
course.addElement("PHP");
lbl=new JLabel("Select Course: ");
li=new JList(course);
btn=new JButton("Click");
lbl.setBounds(40, 40, 100, 40);
li.setBounds(40, 80, 80, 120);
btn.setBounds(150, 120, 100, 30);
add(lbl);
add(li);
add(btn);
btn.addActionListener(this);
setLayout(null);
setSize(400,300);
setTitle("Radio Button");
setVisible(true);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
}
public void actionPerformed(ActionEvent ae)
{
String msg = "";
int selectedIndices[] = li.getSelectedIndices();
for (int j = 0; j < selectedIndices.length; j++) {
String elem =(String) li.getModel().getElementAt(selectedIndices[j]);
msg += "\n" + elem;
}
JOptionPane.showMessageDialog(this,msg);
}
}
public class ListExample
{
public static void main(String[] args)
{
ListEx list=new ListEx();
}
}
Output
The code above will display :
.png)
Example to add elements in a Jlist from JTextField and performing different operations on it.
import java.awt.BorderLayout;
import java.awt.Container;
import java.awt.FlowLayout;
import java.awt.GridLayout;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.*;
import javax.swing.JFrame;
import javax.swing.JTextField;
public class ListExample extends JFrame implements ActionListener{
JTextField txt1;
JList com;
JButton btnadd,btnshow,btnrem,btnremall,btnindex;
Container c;
JPanel p1,p2,p3;
DefaultListModel model;
public ListExample()
{
setLayout(null);
txt1= new JTextField();
model = new DefaultListModel();
com = new JList(model);
btnadd = new JButton("Add");
btnshow = new JButton("Show");
btnrem = new JButton("Remove");
btnremall = new JButton("Remove All");
btnindex = new JButton("Show Index");
c = getContentPane();
c.setLayout(new FlowLayout());
c.setVisible(true);
p1= new JPanel(new GridLayout(1, 2));
p2=new JPanel(new BorderLayout());
p3= new JPanel(new GridLayout(5, 1));
btnadd.addActionListener(this);
btnshow.addActionListener(this);
btnrem.addActionListener(this);
btnremall.addActionListener(this);
btnindex.addActionListener(this);
p2.add("North",txt1);
p2.add("Center",com);
p3.add(btnadd);
p3.add(btnshow);
p3.add(btnindex);
p3.add(btnrem);
p3.add(btnremall);
setDefaultCloseOperation(EXIT_ON_CLOSE);
p1.add(p2);
p1.add(p3);
c.add(p1);
}
public void actionPerformed(ActionEvent e) {
String str=txt1.getText().trim();
if(e.getActionCommand()=="Add")
{
model.addElement(str);
txt1.setText("");
txt1.requestFocus();
}
else if(e.getActionCommand()=="Show")
{
JOptionPane.showMessageDialog(rootPane,"You Have Selected:"+com.getSelectedValue().toString());
}
else if(e.getActionCommand()=="Remove")
{
int i= com.getSelectedIndex();
model.remove(i);
}
else if(e.getActionCommand()=="Remove All")
{
model.clear();
}
else if(e.getActionCommand()=="Show Index")
{
Integer i=com.getSelectedIndex();
txt1.setText(i.toString());
}
}
public static void main(String []args)
{
ListExample list=new ListExample();
list.setVisible(true);
list.setSize(400, 400);
}
}
Output
The code above will display :

Example to add elements in a Jlist from JTextField and do operations to move elements from one list to another.
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.*;
import javax.swing.JFrame;
public class ListExample extends JFrame implements ActionListener{
JList list1,list2;
DefaultListModel model1,model2;//model required to add elements to JList
JButton btnadd,btn1,btn2,btn3,btn4;
Container c;
JPanel p1,p2,p3,p4,p5,p6;
JTextField txt1;
String [] str ={"check","test"};
public ListExample()
{
setLayout(null);
txt1= new JTextField(10);
model1 = new DefaultListModel();
model2 = new DefaultListModel();
list1= new JList(model1);
list2 = new JList(model2);
btnadd = new JButton("Add");
btn1 = new JButton(">");
btn2 = new JButton("<");
btn3 = new JButton(">>");
btn4 = new JButton("<<");
p1 = new JPanel();
p2 = new JPanel();
p3 = new JPanel();
p4 = new JPanel();
p5 = new JPanel();
p6 = new JPanel();
btnadd.addActionListener(this);
btn1.addActionListener(this);
btn2.addActionListener(this);
btn3.addActionListener(this);
btn4.addActionListener(this);
p2.setLayout(new FlowLayout());
p2.add(txt1);
p2.add(btnadd);
p3.setLayout(new FlowLayout());
p3.add(list1);
p4.setLayout(new GridLayout(4, 1));
p4.add(btn1);
p4.add(btn2);
p4.add(btn3);
p4.add(btn4);
p5.setLayout(new FlowLayout());
p5.add(list2);
p6.setLayout(new FlowLayout());
p6.add(p3);
p6.add(p4);
p6.add(p5);
p1.setLayout(new BorderLayout());
p1.add("North",p2);
p1.add("Center",p6);
c=getContentPane();
c.setLayout(new FlowLayout());
c.setVisible(true);
c.add(p1);
setDefaultCloseOperation(EXIT_ON_CLOSE);
}
@Override
public void actionPerformed(ActionEvent e) {
String str=txt1.getText().trim();
if(e.getActionCommand()=="Add")
{
model1.addElement(str);
txt1.setText("");
txt1.requestFocus();
}
else if(e.getActionCommand()==">")
{
int i = list1.getSelectedIndex();
model2.addElement(model1.getElementAt(i).toString());
model1.remove(i);
}
else if(e.getActionCommand()=="<")
{
int i = list2.getSelectedIndex();
model1.addElement(model2.getElementAt(i).toString());
model2.remove(i);
}
else if(e.getActionCommand()==">>")
{ for(int i=0;i<model1.getSize();i++)
{
model2.addElement(model1.getElementAt(i));
}
model1.clear();
}
else if(e.getActionCommand()=="<<")
{
for(int i=0;i<model2.getSize();i++)
{
model1.addElement(model2.getElementAt(i));
}
model2.clear();
}
}
public static void main(String[] args)
{
ListExample list=new ListExample();
list.setVisible(true);
list.setSize(500, 500);
}
}
Output
The code above will display :
