What is Doubly linked list?
The linked list which has two link parts one is previous and other is next and one part is the data part. In doubly linked list traversing can be done from any of the two sides previous or next.
Doubly linked list
Prev : Prev is a pointer which points to the
Previous Node.
Next: Next is a pointer which points to the Next
Node.
Insertion in doubly linked list
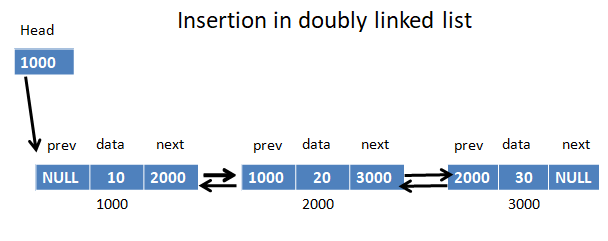
Deletion in Doubly Linked List
Delete the given Node
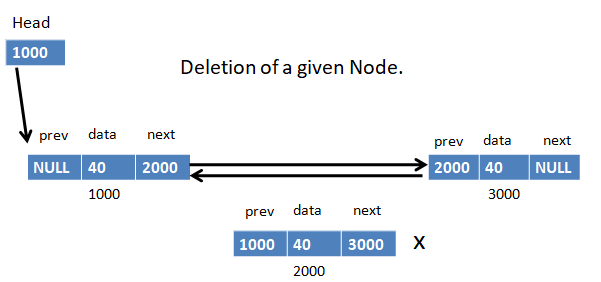
Code for Doubly Linked List
#include<iostream>
using namespace std;
class Node
{
public:
Node *left;
int data;
Node *right;
};//end of class
Node *head=NULL,*nextnode=NULL,*tail=NULL;
void createNode(int value)
{
Node *ptr;
ptr=new Node();
ptr->left=NULL;
ptr->data=value;
ptr->right=NULL;
//node created
if(head==NULL)
{
head=ptr;
nextnode=ptr;
tail=ptr;
}
else{
ptr->left=nextnode;
nextnode->right=ptr;
nextnode=ptr;
tail=ptr;
}
cout<<endl<<"New node created";
}//end of function
void traversLeftToRight()
{
Node *ptr;
ptr=head;
cout<<endl<<"Traversing Left to Right"<<endl;
while(ptr!=NULL)
{
cout<<ptr->data<<" ";
ptr=ptr->right;
}
}//end of function
void traversRightToLeft()
{
Node *ptr;
ptr=tail;
cout<<endl<<"Traversing Right to Left"<<endl;
while(ptr!=NULL)
{
cout<<ptr->data<<" ";
ptr=ptr->left;
}
}//end of function
void deleteAtEnd()
{
Node *ptr;
ptr=tail->left;
ptr->right=NULL;
delete tail;
tail=ptr;
nextnode=ptr;
cout<<endl<<"Last Node Deleted...";
}//end of function
void deleteAtBeg()
{
Node *ptr;
ptr=head->right;
ptr->left=NULL;
delete head;
head=ptr;
cout<<endl<<"First Node Deleted...";
}//end of function
void deleteAtMid(int value)
{
Node *ptr,*prev;
int found=0;
ptr=head;
while(ptr!=NULL)
{
if(ptr->data==value)
{
Node *temp;
temp=ptr->right;
prev->right=temp->left;//ptr->right;
temp->left=prev;
found=1;
cout<<endl<<"Node deleted...";
break;
}
prev=ptr;
ptr=ptr->right;
}//end of while
if(found==0)
{
cout<<endl<<"Node not found...";
}
}//end of function
int main()
{
createNode(10);
createNode(20);
createNode(30);
createNode(40);
traversLeftToRight();
traversRightToLeft();
deleteAtEnd();
traversLeftToRight();
traversRightToLeft();
deleteAtBeg();
traversLeftToRight();
traversRightToLeft();
createNode(10);
createNode(40);
traversLeftToRight();
traversRightToLeft();
cout<<endl<<"Deleting node of value 10";
deleteAtMid(10);
traversLeftToRight();
traversRightToLeft();
}//end of main