Arrays.
A technique which is used to store multiple values but of same data type. In array contigious memory locations are formed and every memory location is assigned with unique index number starting from 0.
Insertion in an Array.
Insertion in an array means insert an element in an array at a given location.
Inserting element 25 at index 2 in array.
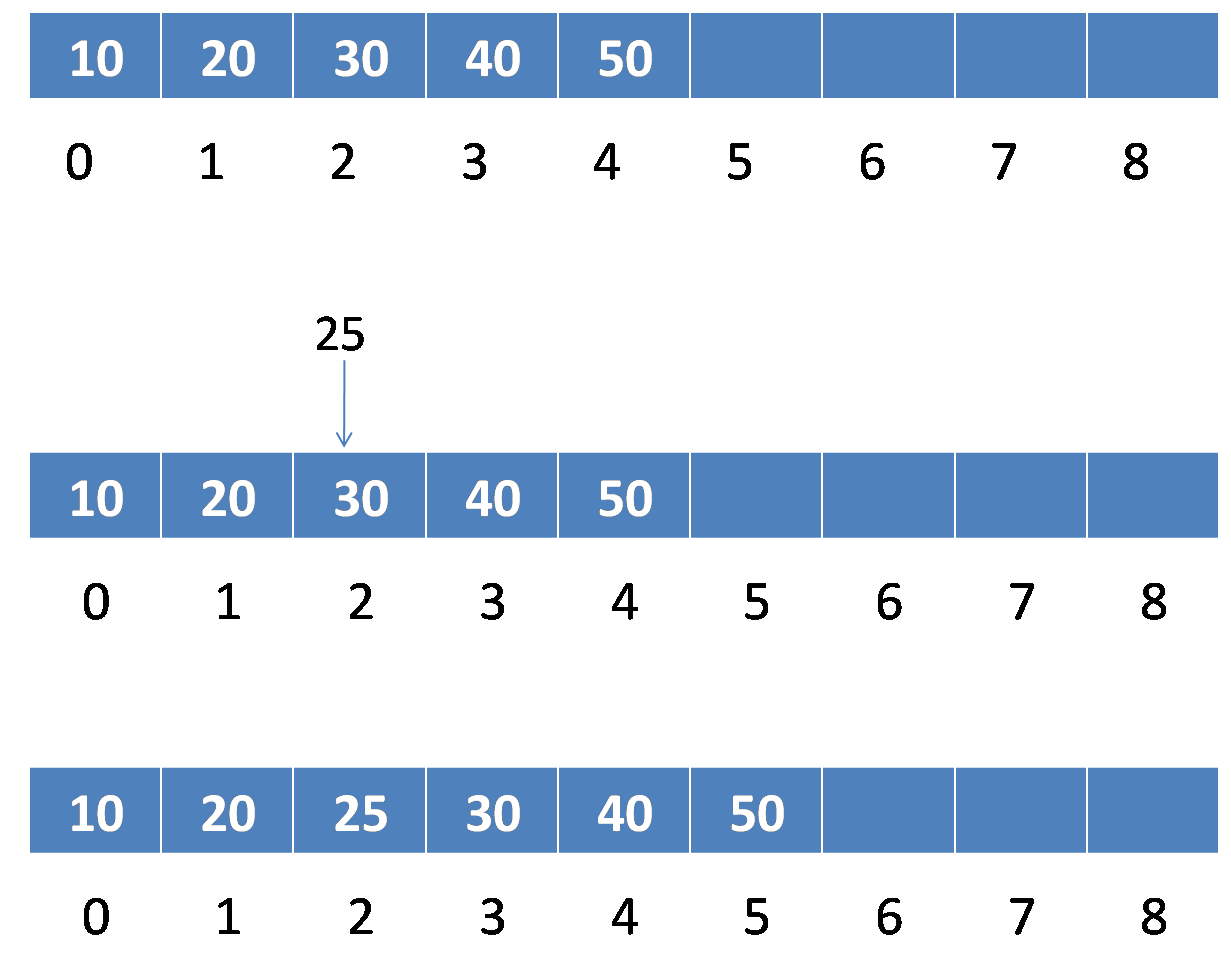
Algorithm of insertion in an Array
INSERT(A,N,LOC,ITEM) [A is an array, N number of elements, LOC is location]
Step1. START
Step2. Repeat Step 3 For I <- N to I ≥ LOC, I <- I - 1
Step3. A[I+1] <- A[I] [Moves elements with index I to Right by 1]
Step4. A[LOC] <- ITEM
Step5. N <- N+1 [Reset size to N+1]
Step6. END
Program of Insertion in an Array.
#include <stdio.h>
#include<conio.h>
void insert(int array[],int *n,int item,int loc)
{
int i;
for(i=*n-1;i>=loc;i--)
{
array[i+1]=array[i];
}
array[loc] = item;
*n=*n+1;
}
void main()
{
int array[50], loc, i, n, item;
printf("Enter number of elements in the array\n");
scanf("%d", &n);
printf("Enter %d elements\n", n);
for (i = 0; i < n; i++)
{
scanf("%d", &array[i]);
}
printf("Please enter the location where you want to insert an item\n");
scanf("%d", &loc);
printf("Please enter the item\n");
scanf("%d", &item);
loc=loc-1;
insert(array,&n,item,loc);
printf("Resultant array is\n");
for (i = 0; i < n; i++)
{
printf("%d\n", array[i]);
}
getch();
}//end of main