Stack Data Strucutre
It is a linear data structure in which elements are arranged in Last In First Out (LIFO) order. It means we can add an element only at the top of the stack and remove an element from the top of the stack. But, we can't add or remove element in between the stack.
Operations performed in Stack.
push()
pop()
peek()
Push operation in Stack
Push operation in stack simply means to insert an elment at the top of the stack..
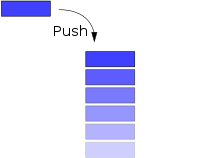
Algorithm of push operation in Stack
Push Algorithm
Step 1. Start
Step 2. TOP := 1 [initialize TOP to 1]
Step3. If TOP := MAXSIZE-1
Write “Overflow : Stack is full” and Exit
[End of If]
Step 4: TOP :=TOP+1
Step 5: Stack[TOP] :=ITEM
Step 6: End
Pop operation in Stack
Pop operation in stack simply means to delete an elment from the top of the stack..

Algorithm of pop operation in Stack
Pop Algorithm
Step1: Start
Step 2: If TOP := 0
Print “Underflow: Stack is empty” and Exit
[End of if]
Step 3: ITEM :=Stack[TOP]
Step 4: TOP := TOP-1
Step 5: ITEM [Deleted element]
Step 6: End
Peek operation in Stack
Peek operation in stack simply means to just look an elment at the top of the stack..

Algorithm of peek operation in Stack
Peek Algorithm
Step1: Start
Step 2: If TOP := 0
Print “Underflow: Stack is empty” and Exit
[End of if]
Step 3: ITEM: =Stack [TOP]
Step 4: ITEM [Top element]
Step 5: End
Program of Stack Performing push(), pop() and peek() by using array.
#include<stdio.h>
int stack[100],size=100,top=-1;
void push(int val)
{
if(top>=size-1)
printf("Overflow: Stack is full \n");
else
{
top++;
stack[top]=val;
}
}
void pop()
{
if(top<=-1)
{
printf("Stack Underflow \n");
}
else
{
printf("The popped element = %d ",stack[top]);
top--;
}
}
void peek()
{
if(top<=-1)
{
printf("Stack Underflow \n");
}
else
{
printf("The topmost element = %d \n",stack[top]);
}
}
void show()
{
if(top>=0)
{
printf("Stack Elements are\n");
for(int i=0;i<=top;i++)
printf("%d ",stack[i]);
}
else
{
printf("Stack is Empty");
}
}
void main()
{
int choice,val;
do
{
printf("\nMenu");
printf("\n1. PUSH Element");
printf("\n2. POP ");
printf("\n3. PEEK");
printf("\n4. SHOW Stack");
printf("\n5.Exit");
printf("\nEnter your choice 1 to 5=");
scanf("%d",&choice);
switch (choice)
{
case 1:
printf("Enter value to be Pushed=");
scanf("%d",&val);
push(val);
break;
case 2:
pop();
break;
case 3:
peek();
break;
case 4:
show();
break;
case 5:
exit(0);
break;
default:
printf("Invalid choice !");
break;
}
}while (choice<=4);
}